This is a collection of how to increase your coding speed in visual studio. I will write this based on Visual Studio 2017:
Search:
You have the ability to search for everything you can imagine with [(ctrl + q)]. With the escape-key you can go one step back with the cursor into your code.
Bookmarks:
With bookmarks you can go to set a reminder to review this code-part later by pressing [(ctrl + k) + (ctrl + k)]. This works like a debugger-point. By pressing [(ctrl + w) + (ctrl + k)] you can see all bookmarks. With the menu-key (right next to the right windows-key) you can rename or delete a bookmark. With just hitting enter you can go and visit a place that you marked with a bookmark.
Code snippets:
As well there are a lot of code snippets that will increase your coding speed. Here are some examples:
- ctor: constructor
- prop: property
- propfull: property with full get and set methods
- wl: write line
- equals: overriding object.Equals and object.GetHashCode function
- try: try block
- tryf: try block with a finally
- for: a for loop
- forr: a for loop which will decrease
- ctor: constructor
- foreach: foreach loop; if you created a list right before doing this it will auto-suggest the name of the list that you created
- while: while-loop
- do: do-while loop
- …
Auto-Format:
To auto-format your code you can use the shortcut [(ctrl + k) + (ctrl + f)]. Another opportunity is to download the Microsoft “Productivity Power Tools“. After installing you have to go to Options -> Productivity Power Tools -> PowerCommands:General and make sure that “Format document on save” is set. Now you just have to save the file ad it will auto-format everything. As well it will remove all of the using-statements that are not used right now.
Switching Tabs:
As well you should use the keyboard to switch the tabs in Visual Studio. For this you can use the shortcut [(ctrl + tab)]. To go to the last tab you can go with [(ctrl + shitft + F6)]. To close a tab you can use [(ctrl + F4)].
Navigation
To navigate through your project I recommend you to use the shortcut [(ctrl + ;)]. This will open an dialog which will give you the opportunity to search for everything that you need. You can search for a class, a method, a file, …
Concentration to the right code
To bring you into the code you can switch between full-screen and the normal view with [(shift + alt + enter)]. To collapse code blocks you can use [(ctrl + m) + (ctrl + m)]. This works on every level (class, method, …).
Let Visual Studio work for you
In Visual Studio there is another godlike opportunity to avoid coding. If you have any part that is underlined you can go there and use [(alt + enter)] to have some options that will suggest you to finish your code correctly:
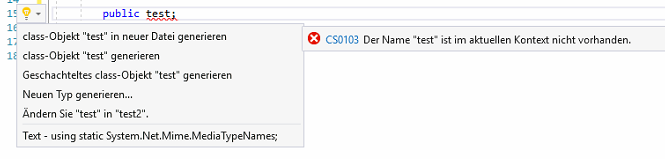
You must be logged in to post a comment.